Bayesian Optimization in Trading
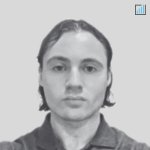
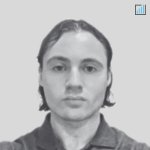
Bayesian optimization is a probabilistic model-based approach for optimizing objective functions that are expensive to evaluate.
This technique is handy in trading strategies where backtesting and parameter tuning can be computationally intensive.
This strategy is useful for more technically-oriented traders of all stripes – from scalpers and day traders through longer-term position traders.
Key Takeaways – Bayesian Optimization in Trading
- Bayesian optimization enables efficient exploration of high-dimensional trading strategy parameter spaces, with the intent to maximize profitability.
- It intelligently balances exploration and exploitation – i.e., adapting to new market conditions and avoiding local optima.
- By incorporating prior knowledge and historical data, Bayesian optimization can help identify robust and consistent trading strategies.
- Bayesian logic mimicks how markets operate in the real-world – updating our beliefs as new information comes in.
Here’s a step-by-step example of applying Bayesian optimization to optimize a trading strategy:
Step 1: Define the Trading Strategy and Parameters
Trading Strategy
Let’s consider a simple moving average crossover strategy where we have two parameters:
- the short-term moving average window (e.g., 5 days) and
- the long-term moving average window (e.g., 20 days)
Parameters to Optimize:
- Short-term moving average window (5-50 days)
- Long-term moving average window (50-200 days)
Step 2: Define the Objective Function
The objective function should reflect the performance of the trading strategy.
Common objectives include maximizing:
- the Sharpe ratio
- total return, or
- minimizing the maximum drawdown
For this example, we will optimize for the Sharpe ratio.
Step 3: Set Up the Bayesian Optimization Framework
Surrogate Model
Use a Gaussian Process (GP) as the surrogate model to approximate the objective function.
Acquisition Function
Use an acquisition function such as Expected Improvement (EI) or Upper Confidence Bound (UCB) to guide the search for the optimum.
Step 4: Initialize the Optimization Process
Start with a few randomly selected points in the parameter space to initialize the Gaussian Process.
Step 5: Iteratively Update the Model
Fit the GP Model
Train the GP model on the initial set of parameters and their corresponding objective values.
Optimize Acquisition Function
Find the next set of parameters by optimizing the acquisition function.
Evaluate Objective Function
Backtest the trading strategy with the new set of parameters and compute the Sharpe ratio.
Update the GP Model
Incorporate the new parameters and their objective value into the GP model.
Step 6: Convergence
Continue the iterations until convergence criteria are met, such as a maximum number of iterations or a threshold for improvement in the objective function.
Code Example of Bayesian Optimization
Here’s some example Python code for Bayesian optimization.
import numpy as np from skopt import gp_minimize from skopt.space import Integer from skopt.utils import use_named_args # Define parameter space space = [Integer(5, 50, name='short_window'), Integer(50, 200, name='long_window')] # Define the objective function @use_named_args(space) def objective(params): short_window, long_window = params # Example backtest function (replace with actual backtest logic) sharpe_ratio = backtest_strategy(short_window, long_window) return -sharpe_ratio # Minimize the negative Sharpe ratio # Example backtest function (simplified) def backtest_strategy(short_window, long_window): # This function should implement the actual backtesting logic # For simplicity, let's use a mock Sharpe ratio mock_sharpe_ratio = np.random.uniform(0, 2) return mock_sharpe_ratio # Perform Bayesian Optimization res_gp = gp_minimize(objective, space, n_calls=50, random_state=0) # Output the best parameters print(f"Best parameters: Short Window: {res_gp.x[0]}, Long Window: {res_gp.x[1]}") print(f"Best Sharpe Ratio: {-res_gp.fun}")
Application in Real-world Trading
Data Collection
Gather historical price data for the asset(s) of interest.
Preprocessing
Clean the data and calculate necessary indicators (e.g., moving averages).
Backtesting Framework
Implement a robust backtesting framework that can simulate trades and calculate performance metrics.
Bayesian Optimization Loop
Integrate the backtesting framework into the Bayesian optimization loop as shown in the example.
Optimization Execution
Run the optimization process to find the optimal parameters.
Strategy Deployment
Deploy the strategy with the optimized parameters in a live trading environment. Be sure to continuously monitor and update as needed.
This approach gives an efficient search for optimal trading strategy parameters, leveraging Bayesian optimization to handle expensive and noisy objective functions effectively.
Advantages
- Efficiency – Requires fewer evaluations of the objective function compared to traditional methods.
- Uncertainty Quantification – Provides uncertainty estimates that guide the search process.
- Flexibility – Can be applied to a wide range of optimization problems.
Limitations
- Scalability – May not scale well with high-dimensional problems due to the computational complexity of Gaussian Processes.
- Complexity – Implementation and understanding of the method can be complex.
Bayesian optimization is a unique analysis method for optimizing expensive black-box functions – balancing exploration and exploitation to efficiently find optimal solutions.